Best practice alignment of user managment
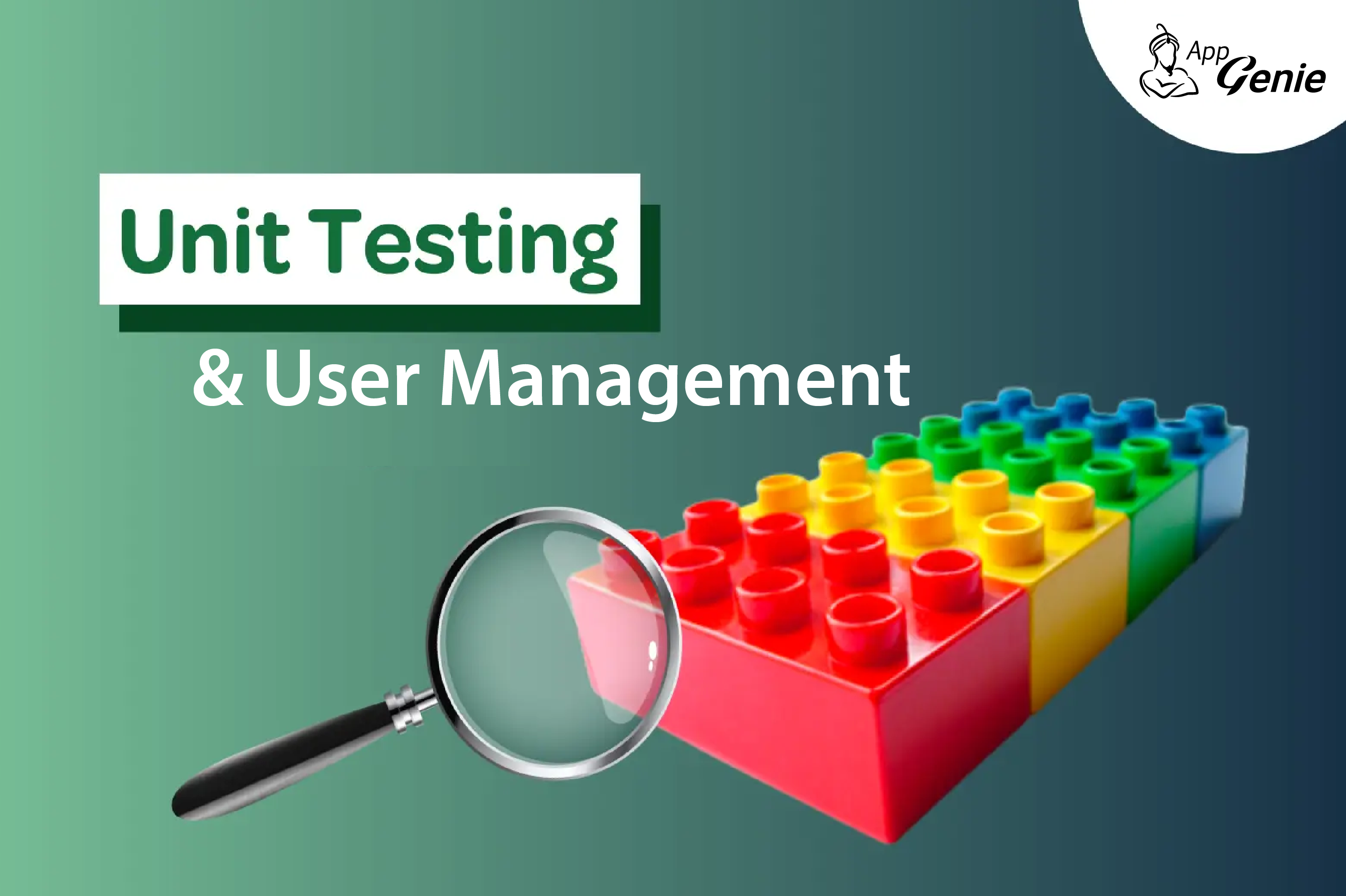
Unit Testing and User Management
This month we’re diving back into Salesforce coding and working through some best practice unit test patterns. Whether you’re new to these patterns or you’ve been around the block, there’s always room to make our code more robust, secure, and reflective of production realities.
This is the first in a series of two articles. This one defines why we need a solution that is better than what we currently have, so please grab a coffee, and let’s roll up our sleeves.
Setting the Scene
Let’s start by defining an LWC controller that performs a simple update on the Account object. In any Salesforce organisation, this kind of operation is common—and a perfect example to expose common pitfalls. Here’s a minimal Apex class exposed to LWC via an @AuraEnabled method:
public with sharing class AccountUpdateController {
@AuraEnabled
public static void updateAccount(Id accountId, String newName) {
// Querying the account record (assumes account exists)
Account acc = [SELECT Id, Name FROM Account WHERE Id = :accountId LIMIT 1];
acc.Name = newName;
update acc;
}
}
At first glance, this is straightforward. However, if you were to run a Salesforce scanner on it, you’d probably see several red flags—hardcoded assumptions, potential security issues, and the lack of error handling. Were going to ignore those - but you shouldn't! Now let’s see how our unit test can be built, then we’ll iterate.
The initial approach is often to simply write a test that runs as the current user. It might look like this:
@isTest
private class TestAccountUpdateController {
static testMethod void testUpdateAccount_DefaultUser() {
// Create a new account record
Account acc = new Account(Name = 'Old Name');
insert acc;
// Call our update method in the current system context
AccountUpdateController.updateAccount(acc.Id, 'New Name');
// Validate the update
Account updated = [SELECT Name FROM Account WHERE Id = :acc.Id];
System.assertEquals('New Name', updated.Name);
}
}
This test will pass—but it’s fundamentally flawed. The user running this test is the one executing the test method. This is a security red flag, because it doesn’t simulate a real-world scenario where a specific business user with defined privileges is running the code. The result? Your test might pass under ideal conditions but fail if executed using a different user context or worse the unit test pass but the business functionality fail in a production environment
Introducing a Test User for Better Context
To better simulate production conditions, we should execute our tests using a dedicated test user. This allows us to mimic the privilege boundaries that exist in your organisation. Here’s an improved version using System.runAs:
@isTest
private class TestAccountUpdateController {
static testMethod void testUpdateAccount_RunAsUser() {
// Create a test user (assuming a standard user profile has appropriate privileges)
Profile p = [SELECT Id FROM Profile WHERE Name = 'Standard User' LIMIT 1];
User testUser = new User(
Alias = 'tuser',
Email = 'tuser@test.com',
EmailEncodingKey = 'UTF-8',
LastName = 'User',
LanguageLocaleKey = 'en_US',
LocaleSidKey = 'en_US',
ProfileId = p.Id,
TimeZoneSidKey = 'America/New_York',
UserName = 'tuser@test.com'
);
insert testUser;
// Set up test data
Account acc = new Account(Name = 'Old Name');
insert acc;
// Execute under the context of testUser
System.runAs(testUser) {
AccountUpdateController.updateAccount(acc.Id, 'New Name');
}
// Validate the update
Account updated = [SELECT Name FROM Account WHERE Id = :acc.Id];
System.assertEquals('New Name', updated.Name);
}
}
This is much better — now we’re simulating a real business user. But wait… there’s more...
Handling Negative Test Cases: When Privileges Are Missing
In robust testing, you must not only confirm that your code works under ideal conditions but also that it fails gracefully when things go wrong. For instance, what happens when a user without the necessary privileges tries to update an account? Let’s simulate that by creating a test user with a read-only profile:
@isTest
private class TestAccountUpdateController_Negative {
static testMethod void testUpdateAccount_NoPrivileges() {
// Create a test user with a read-only profile (simulate insufficient privileges)
Profile readOnlyProfile = [SELECT Id FROM Profile WHERE Name = 'Read Only' LIMIT 1];
User readOnlyUser = new User(
Alias = 'roUser',
Email = 'rouser@test.com',
EmailEncodingKey = 'UTF-8',
LastName = 'User',
LanguageLocaleKey = 'en_US',
LocaleSidKey = 'en_US',
ProfileId = readOnlyProfile.Id,
TimeZoneSidKey = 'America/New_York',
UserName = 'rouser@test.com'
);
insert readOnlyUser;
// Set up test data
Account acc = new Account(Name = 'Old Name');
insert acc;
// Execute the update under the read-only user's context
try {
System.runAs(readOnlyUser) {
AccountUpdateController.updateAccount(acc.Id, 'New Name');
}
// If we reach here, the update did not throw an exception as expected
System.assert(false, 'Expected an exception due to insufficient privileges.');
} catch (Exception e) {
// Optionally assert that the exception message contains something indicative of a privilege error
System.assert(
e.getMessage().contains('insufficient privileges') ||
e.getMessage().contains('required access'),
'Expected a privilege-related error message.'
);
}
}
}
By testing both positive and negative scenarios, we ensure that our code behaves as expected, no matter what user is behind the wheel.
Beyond the Basics: Persona Management
As systems grow in complexity, simply testing “happy paths” and “sad paths” isn’t enough. In larger, more protected environments, you’ll have a
multitude of user personas - each with their own unique permissions, data access, and operational constraints.
For instance, you might have:
-
The Business User:
Has permissions to update and view their own data. -
The Administrator:
Can override many restrictions but must still adhere to audit logging. -
The External Partner:
Has limited access to only a subset of records and fields.
Each of these personas should be represented in your tests. One way to manage this complexity is by abstracting user creation and test data into a Test Data Factory.
Test Data Factory: A Recommended Pattern
Using a Test Data Factory pattern, you centralise your logic for creating test data. This not only reduces duplication in your tests but also helps maintain consistency. Here’s a simplified example:
public class TestDataFactory {
public static User createTestUser(String profileName, String uniqueIdentifier) {
Profile p = [SELECT Id FROM Profile WHERE Name = :profileName LIMIT 1];
User u = new User(
Alias = uniqueIdentifier.substring(0, 5),
Email = uniqueIdentifier + '@test.com',
EmailEncodingKey = 'UTF-8',
LastName = 'Test',
LanguageLocaleKey = 'en_US',
LocaleSidKey = 'en_US',
ProfileId = p.Id,
TimeZoneSidKey = 'America/New_York',
UserName = uniqueIdentifier + '@test.com'
);
insert u;
return u;
}
public static Account createAccount(String accountName) {
Account acc = new Account(Name = accountName);
insert acc;
return acc;
}
}
And then refactor your test cases:
@isTest
private class TestAccountUpdateController_WithFactory {
static testMethod void testUpdateAccount_WithFactory() {
// Create test user via factory
User testUser = TestDataFactory.createTestUser('Standard User', 'standardUser1');
// Create an account record via factory
Account acc = TestDataFactory.createAccount('Old Name');
System.runAs(testUser) {
AccountUpdateController.updateAccount(acc.Id, 'New Name');
}
Account updated = [SELECT Name FROM Account WHERE Id = :acc.Id];
System.assertEquals('New Name', updated.Name);
}
}
This approach not only makes your tests cleaner but also provides a single point of truth for test data creation. It becomes invaluable when your test requirements evolve with your application’s complexity. But it still is fundamentally flawed due to Salesforce platform limits.
Dynamically Generating Unique User Identifiers
We’ll modify our factory method to generate a unique email and login name for every test user. By appending a timestamp (or a unique token), we avoid collisions. This method can also accept parameters for locale, time zone, and language, allowing us to simulate different user environments.
public class TestDataFactory {
/**
* Creates a test user with a dynamic unique email and locale/timezone settings.
* @param profileName The name of the profile to use.
* @param uniqueIdentifier A base string to help identify this user.
* @param locale The locale for the user, e.g., 'en_US', 'fr_FR', etc.
* @param timeZone The timezone for the user, e.g., 'America/New_York'.
* @param languageLocaleKey The language locale key, e.g., 'en_US', 'fr'.
*/
public static User createTestUser(String profileName, String uniqueIdentifier, String locale, String timeZone, String languageLocaleKey) {
// Retrieve the profile ID dynamically
Profile p = [SELECT Id FROM Profile WHERE Name = :profileName LIMIT 1];
// Append a timestamp to avoid collisions
String uniqueSuffix = String.valueOf(DateTime.now().getTime());
String uniqueEmail = uniqueIdentifier + '_' + uniqueSuffix + '@test.com';
User u = new User(
Alias = uniqueIdentifier.substring(0, Math.min(uniqueIdentifier.length(), 5)),
Email = uniqueEmail,
EmailEncodingKey = 'UTF-8',
LastName = 'Test',
LocaleSidKey = locale,
LanguageLocaleKey = languageLocaleKey,
ProfileId = p.Id,
TimeZoneSidKey = timeZone,
UserName = uniqueEmail
);
insert u;
return u;
}
public static Account createAccount(String accountName) {
Account acc = new Account(Name = accountName);
insert acc;
return acc;
}
}
Testing Multiple Personas in a Single Test Run
Now that we have a more flexible test data factory, let’s design a test that iterates through a list of user personas. This approach ensures we test every edge—whether it’s a standard business user, a read-only user, or even a user with a custom profile and locale. We’ll simulate positive and negative outcomes based on user permissions.
@isTest
private class TestAccountUpdateController_MultiPersona {
static testMethod void testUpdateAccount_MultipleUsers() {
// Define various user personas with differing profiles and locale settings.
List
Dynamic User Creation: By dynamically generating unique email addresses and usernames, we avoid collisions in concurrent test environments.
Diverse Locales & Formats: Accepting parameters for locale, timezone, and language means we can easily simulate a global user base with custom datetime or formatting needs.
Comprehensive Testing Across Personas: Iterating through a list of different user profiles allows us to verify that our code behaves correctly across a spectrum of business conditions—from fully privileged to read-only access.
This enhancement not only makes your tests more robust in a concurrent environment but also ensures you’re covering the nuanced behaviors that come with supporting multiple user personas.
Key Takeaways:
-
Dynamic User Creation:
By dynamically generating unique email addresses and usernames, we avoid collisions in concurrent test environments. -
Diverse Locales & Formats:
Accepting parameters for locale, timezone, and language means we can easily simulate a global user base with custom datetime or formatting needs. -
Comprehensive Testing Across Personas:
Iterating through a list of different user profiles allows us to verify that our code behaves correctly across a spectrum of business conditions—from fully privileged to read-only access.
Wrap-up
In this article, we took a deep dive into Salesforce unit testing, focusing on best practices around user management and testing strategies.
In particular we addressed the pitfalls of hard-coded values by dynamically generating unique emails and usernames, and we expanded our tests to
loop through multiple personas with varying locale settings, ensuring our tests hold up in concurrent and global scenarios.
This comprehensive approach not only strengthens our unit tests but also ensures that they accurately reflect the diversity of user contexts
in production environments.
However even with this comprehensive approach there there’s still a couple of problems that can impact us in a Production environment - stay tuned
for the next article where we'll explore even more advanced techniques to tackle the challenges of testing in complex, protected Salesforce
ecosystems.
Happy coding, and may your tests always pass!