Git History
Git (Global Information Tracker) is a distributed version control system that was created by Linus Torvalds in 2005. Since its release, git has become the leading version control system in the software development world due to its speed, reliability, and flexibility.
Git is a free and open source distributed version control system designed to handle everything from small to very large projects with speed and efficiency. Git has a tiny footprint with lightning fast performance. It outclasses SCM tools like Subversion, CVS, Perforce, and ClearCase with features like cheap local branching, convenient staging areas, and multiple workflows.
Whether you're working on a small project or a large-scale enterprise application, it's the essential tool that can help you achieve your goals. I doubt there's a developer who hasn't used git by now, so why do people find it so difficult to use!
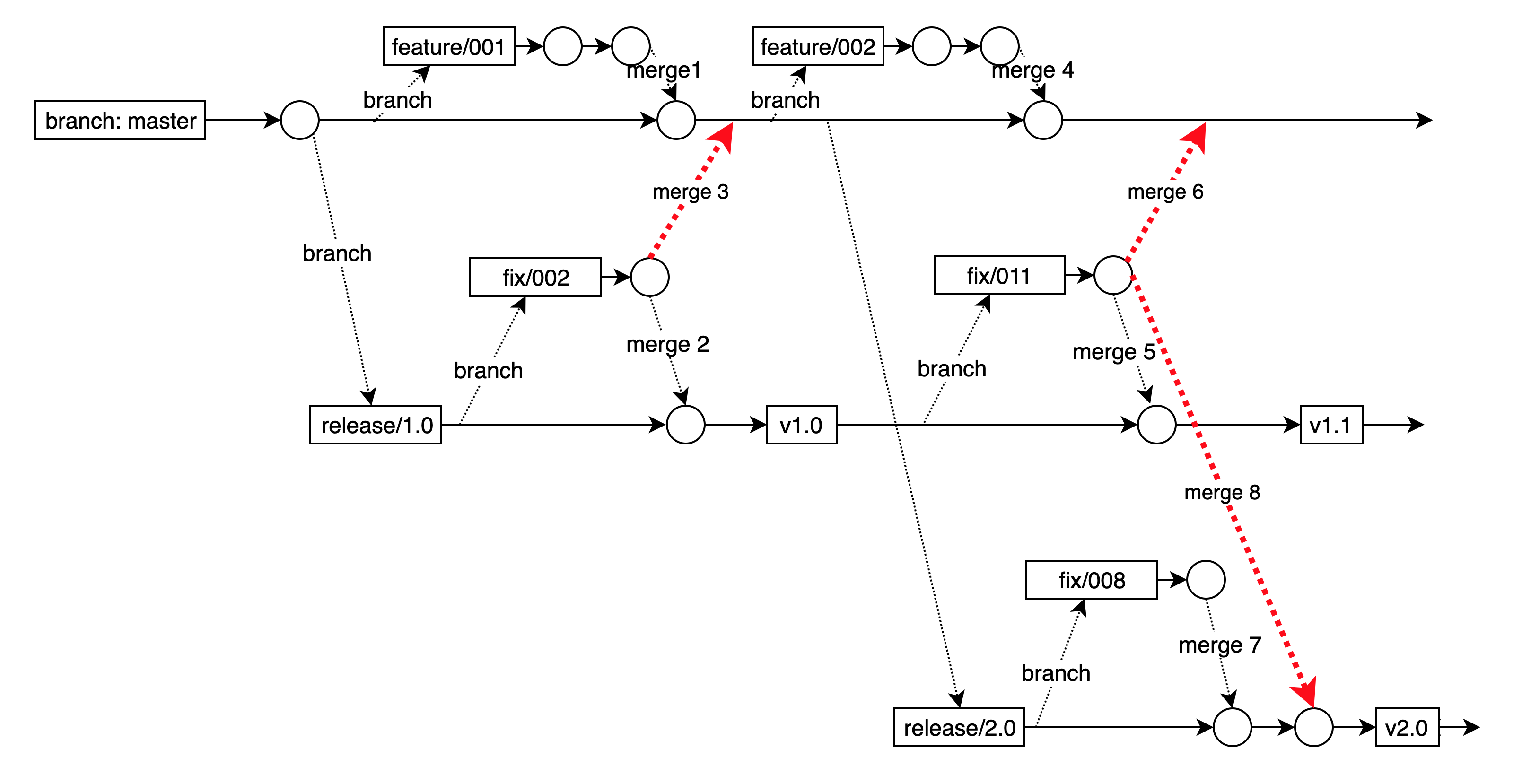
What is it used for?
-
It's like a time machine!
Git allows you to travel back and forth through different versions of your code, you can easily switch between different branches, view the history of changes, and revert to an earlier version of your code when you code yourself into a corner! -
Git is like a gym membership - you don’t use it often, but when you do, it’s a lifesaver.
It acts like a safety net for your code, allowing you to easily revert your code version if something goes wrong. It stores multiple copies as long as you commit often, so you can always retrieve iteration 1051 that worked at 2AM and you haven’t managed to make work since. I have a set of AST code for APEX Class dependencies that worked exactly one ..... another blog post! -
It's like a kitchen gadget - it does so many things, you never knew you needed it until you had it.
Git is your multi-functional tool that can handle a wide range of tasks, including version control, code collaboration, and deployment. It provides features like branching and merging, which make it easy to manage parallel development efforts. Git also integrates with other tools and services, making it an essential part of the modern software development toolkit. -
Git is like a therapist - it listens to all your code’s problems and helps you work through them.
It helps you keep track of all the changes made to your code, allowing you to easily identify and resolve any issues that arise. With Git, you can see a complete history of your code, including who made what changes, when, and why. This makes it easy to collaborate with other developers and troubleshoot problems when they arise.
Who uses it?
Git is a staple tool for software developers, especially for those who work on teams. Whether you're a front-end dev, back-end dev, or full-stack, you'll likely be using Git every day. And it's not just for developers, git is also a crucial tool for DevOps, DevSecOps and SREs who manage code deployments and infrastructure changes. Even project managers and technical leads use git to keep an eye on what's going on in a project. Basically, if you're working on code, git is your best friend!
How do I use it?
While most people use git from a UI, I believe you get the best from it when you get down and dirty and use the command line – but that’s probably because I’m showing my age! I’m assuming your using git bash too, so if you’re not – do so.
Here’s some of my favourite commands and hints. To be honest its also a nice easy place to find this stuff when I need to use them again!
The Basics
I always create the directory structure I want first before cloning a repo. That way I can maintain
my folder structure the way I want – my default is client name then reponame e.g.
source/repos/acme-industries/super-duper-widget
Remember that git is case-sensitive and some of the space handling is a little tricky so keep it simple.
To clone from the repo dev.azure.com\acme-industries make sure you are in the correct directory and then use:
git clone https://username@dev.azure.com/acme-industries/super-duper-widget/_git/reponame
I’m serious about this one, git only works if you have committed your code so do it often.
If you have a good .gitignore in place this is going to be easy, if you don’t how about you use one but
remember – make sure your code compiles and runs first!
git commit -m ‘a message about what has changed’
if you haven't got a decent .gitignore in place you need to collect your changes together individually
git add filename-of-src-file-i-want
or you can wildcard and include everything (git add .) and then remove the stuff you don’t want
git rm filename-of-some-file-I-don’t-want
You’ve committed your code but the remote doesn’t know anything about it unless you Push your
code to the remote, I typically do this twice a day - maybe a little more but always at least once a day
git push
If your branch is not set to the origin repo you will get a message back telling you how to set the remote branch.
If you want to do this manually use:
git push -u origin feature/blinking-button
Before you make your final commit and push of code you need to make sure you have the latest version of everything,
after all there’s more than just you working on stuff right? To do this you need to pull changes into your local branch:
git pull origin master
I’m assuming that we are only dealing with the master branch here but that’s not always the case …
you maybe working on feature/blinking-button that’s part of the next major-release-127 … if so, you need to pull that branch
git pull origin major-release-127
And after you have pushed your changes create a Pull Request!!
How to use - the fun stuff
Okay that stuff is the easy part now lets do some stuff that is fun, I want to:
git log grep ‘cant-find-this-filename’
If you want to see the entire diff of the commit, you can add the -p option to the command:
git log --all -p --grep='cant-find-this-filename'
Add the pretty format option to make it actually readable:
git log --all --pretty=format:"%h %an %ad %s" --grep=’cant-find-this-filename'
git diff branch1 branch2 -- path/to/file
git rebase master
If the hash of the commit hash is 123456 and you want to commit to the current branch:
git cherry-pick 123456
If the source branch is named feature/1234 and you want to commit into the current branch:
git merge feature/1234
git bisect start
Then you will need to identify if you want to keep the current commit if its good
git bisect good
or exclude it from the branch if its bad
git bisect bad
git stash .
git stash apply .
Remove all instances of the file named secret.txt from history i.e. make it go away forever!
git filter-branch --force --index-filter 'git rm --cached --ignore-unmatch secret.txt'
--prune-empty --tag-name-filter cat -- --all
git reset --hard
Whats a reflog? Its the entire history of what has happened in the repo
git reflog
If the commit hash is the latest commit hash in the reflog for that branch
git branch
Sounds great but what about the Bad?
Git does have some limitations so please be aware that while its a fantastic tool there are some use cases that git probably isn't the best tool:
-
Binary files, such as videos or images:
Git cannot identify individual differences in these types of files and their storage in a git repo will cause slow response times. -
File name length and case:
There is a limit on the length of file names that may cause issues so keep your directory structure under control. In addition git is case sensitive so My-super-file is different from my-super-file even if your operating system and IDE think they are the same! -
Line length:
There is a limit on the length of individual lines in a file which can cause problems with very long lines of code, and if you ever exceed it this is a perfect opportunity to refactor and improve code readability and quality! -
Line length:
There is a limit on the length of individual lines in a file which can cause problems with very long lines of code, and if you ever exceed it this is a perfect opportunity to refactor and improve code readability and quality! -
Limited support for renaming and moving files:
Git does not have built-in support for renaming or moving files. A rename or move is treated as a delete and create - this can cause your IDE to have some really funny behaviour. -
Non-linear history: Git's non-linear history can make it difficult to understand and navigate changes to the repository over time. Combine this with the power of branching and merging and people new to version control systems sometimes struggle - hence this post!
And the downright Ugly!
If you've made it this far you're doing great! But here comes the part about git that will
cause you to question your Development or DevOps practices ... lets discuss how git merges branches!
Consider I have the following branches - Branch A (our master), from this I
clone Branch B and C. I then clone Branch E from Branch B and likewise Branch F from Branch C.
I then make a non conflicting change to Branch E and F and merge these back to Branch A.
git checkout A - the merge occurs as per the following
git stash # if there are any local changes in branch A
git merge E
git merge F
git stash pop # if stash was created in step 2
The important part here is that git will perform a diff on each of the branches in this process this can lead to issues due to changes that are identical in branch F and branch A.
If git (correctly) recognises that a file in branch A is changed (in branch E) it will apply this change, if the same file reverts that change in branch F this will be recognised as a change and r evert to the original value.
You think this never happens? Let me discuss how Copado performs merges a release merge, but that's for a later post.
Naming Conventions:
Naming Conventions are incredibly helpful in most repos, here's some suggestions, and don't forget you can create a branch tree just like a directory structure:
-
•
feature - significant development across a team -
•
dev - day to day development -
•
env - environment branch, including all configuration management settings -
•
rel(ease) - merged release candidates -
•
master - your production environment
Congratulations, you made it!
No matter if you're a Software Developer writing some APEX code for Salesforce, a C# Dev smashing out the latest Blazor App, a DevSecOps Engineer spinning EC2's to drive super app #125 or a Release Manager, you need a bare minimum of git skills to be an effective in your respective development and operations team. Git is not scary and you cant avoid it within software development - so why not embrace git and become the Subject Matter Expert!
This is article 1 on GIT, in a series of 3. Please find links to each in the series.